Title: Ethereum code crypto
Ethereum code crypto
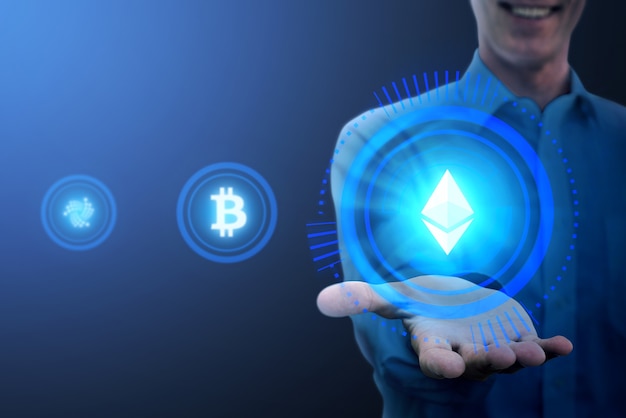
Gas fees on the second-largest blockchain can spike unpredictably. To minimize costs, schedule transactions during low-traffic periods–typically between 1 AM and 5 AM UTC. Tools like Etherscan’s Gas Tracker provide real-time data to time your interactions efficiently.
Developers working with Solidity should prioritize gas-efficient patterns. Replacing loops with mappings, using fixed-size arrays, and avoiding storage operations in loops can cut expenses by up to 30%. Auditing contracts with tools like Slither or MythX helps identify inefficiencies before deployment.
Layer-2 solutions like Arbitrum or Optimism reduce latency and fees by processing transactions off-chain. Migrating dApps to these networks can lower costs by 90% compared to mainnet activity. For high-frequency traders, automated scripts with dynamic fee adjustments ensure optimal execution without manual intervention.
Hardware wallets remain the safest option for storing private keys, but multisig setups add another layer of security. Platforms like Gnosis Safe allow customizable approval thresholds, reducing single-point vulnerabilities. Always verify contract addresses before interacting–scammers often deploy lookalike tokens.
Ethereum Code Crypto: Practical Insights
Use decentralized exchanges (DEXs) like Uniswap or Sushiswap for lower fees compared to centralized platforms. Gas costs fluctuate–check oil-profit.org for real-time network fee tracking before executing transactions.
Smart contract audits prevent exploits. Platforms like Certik and OpenZeppelin verify security, reducing risks in token swaps or yield farming.
Layer-2 solutions (Arbitrum, Optimism) cut transaction costs by 80%. Migrate assets from the mainnet to avoid congestion during peak hours.
Stablecoins (USDC, DAI) minimize volatility in trades. Pair them with high-yield protocols like Aave for consistent returns under 10% APY.
Monitor wallet activity with Etherscan. Revoke unused token approvals to prevent unauthorized access–over $200M was stolen in 2023 due to unchecked permissions.
How to Write and Deploy a Smart Contract with Ethereum Code
Install Node.js and npm, then run npm install -g truffle
to set up the development environment.
Create a new project folder and initialize Truffle with truffle init
to generate the required directories.
Write the contract in Solidity inside the contracts/
folder. Example:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract SimpleStorage {
uint storedData;
function set(uint x) public {
storedData = x;
}
function get() public view returns (uint) {
return storedData;
}
}
Compile the agreement with truffle compile
to generate bytecode and ABI.
Configure network settings in truffle-config.js
, specifying a testnet like Sepolia or Goerli.
Run truffle migrate --network [network_name]
to deploy. Ensure testnet ETH is available in your wallet for gas fees.
Interact with the deployed instance using web3.js or ethers.js:
const contract = new web3.eth.Contract(abi, address);
await contract.methods.set(42).send({from: account});
Verify the agreement on Etherscan by uploading source files and compiler settings.
Debugging Common Errors in Smart Contract Development
Gas estimation failures often occur due to infinite loops or excessive computations. To resolve:
- Check for unbounded loops (e.g., iterating over dynamic arrays).
- Replace loops with mappings where possible.
- Use
require()
to enforce limits on loop iterations.
Reverts with “Out of Gas” usually indicate:
- Complex operations exceeding block gas limits.
- Unoptimized storage writes (each SSTORE costs 20,000 gas).
For “Stack Too Deep” errors:
- Break functions into smaller parts.
- Use structs to group variables.
- Avoid exceeding 16 local variables per function.
Transaction reverts without error messages often mean:
- Missing
payable
modifier on functions receiving native currency. - Failed
require()
orassert()
conditions. - Insufficient funds for transfers.
Debugging tools:
console.log
in Hardhat tests.- Ethers.js error parsing with
error.data
. - Tenderly for visualizing execution paths.
Optimizing Gas Fees for Ethereum Transactions in Your Code
Batch operations to reduce costs. Group multiple actions into a single transaction using contract methods like multicall or custom logic. This minimizes the overhead of repeated execution.
Use cheaper opcodes. Replace SSTORE and SLOAD with alternatives like MSTORE or MLOAD where possible. Storage operations cost up to 20,000 gas, while memory access is under 10.
Optimize data types. Pack variables into smaller slots (e.g., uint128 instead of uint256) when full precision isn’t needed. Structs with tightly packed fields save storage gas.
Leverage events for off-chain data. Emit logs instead of storing non-critical information on-chain. Events cost ~375 gas per topic, far less than persistent storage.
Cache frequently accessed values. Store repeated computations in memory variables to avoid redundant gas consumption from re-execution.
Minimize loops. Unroll small loops manually or use fixed-size arrays to prevent dynamic iteration costs. Each loop cycle adds gas per operation.
Set gas limits dynamically. Estimate required gas before submitting transactions to avoid overpaying. Libraries like ethers.js provide methods for precise estimation.
FAQ:
What is Ethereum Code Crypto?
Ethereum Code Crypto refers to automated trading software designed for Ethereum and other cryptocurrencies. It uses algorithms to analyze market trends and execute trades based on predefined strategies. Some versions claim to offer high accuracy, but users should verify reliability before investing.
Is Ethereum Code a scam?
There is no definitive proof that Ethereum Code itself is a scam, but users should be cautious. Many automated trading platforms make exaggerated claims about profits. Research reviews, check for transparency in fees, and avoid platforms that demand large upfront payments.
How does Ethereum Code work?
The software scans cryptocurrency markets for price movements and trading signals. When it detects a potential opportunity, it can place buy or sell orders automatically. Users typically set risk parameters, such as stop-loss limits, to manage potential losses.
Can beginners use Ethereum Code?
Yes, but beginners should start with caution. The platform may offer a demo mode to practice trading without real money. Learning basic trading principles and understanding risks is important before relying on automated systems.
What are the risks of using Ethereum Code?
Automated trading carries several risks, including technical failures, sudden market crashes, and false signals. No system guarantees profits, and users can lose money. It’s wise to test strategies with small amounts first and avoid investing more than you can afford to lose.
What is Ethereum Code in cryptocurrency?
Ethereum Code refers to automated trading software designed to analyze the crypto market and execute trades on platforms like Ethereum. It uses algorithms to identify trends and make decisions based on predefined rules, aiming to help users trade more efficiently.